Why using Cognito instead of coding it myself?
AWS Cognito offers the developer best practices with login flow, encryption, and easy integration with a range of authentication providers. These features collectively provide a strong foundation for securing user identities and authentication in your application while minimizing the development effort required on your end. AWS is taking care of storing the user credentials in an secure way, you as a developer does not have to think about hashing, salting or also what algorithm to use.
How to integrate Cognito with Elixir?
defmodule YourApp.AWS do
def client() do
client = %AWS.Client{
access_key_id: System.get_env("AWS_ACCESS_KEY_ID"),
secret_access_key: System.get_env("AWS_SECRET_ACCESS_KEY"),
session_token: System.get_env("AWS_SESSION_TOKEN"),
region: "eu-west-1"
}
end
end
Create new User via AWS SDK in Cognito
client_id = "xxx5gvidkg***********"
user_pool_id = "eu-west-1_XXXxEJ***"
AWS.CognitoIdentityProvider.sign_up(Blogex.AWS.client(), %{
ClientId: client_id,
UserPoolId: user_pool_id,
Username: "bmalum_test",
Password: "SecretPass123%$",
UserAttributes: [%{Name: "email", Value: "some.name@example.com"}]
})
Confirm User with Code (if required by Pool Settings)
AWS.CognitoIdentityProvider.confirm_sign_up(Blogex.AWS.client(), %{
Username: "bmalum_test",
ClientId: client_id,
ConfirmationCode: "185931"
})
Sign User in with Cognito:
AWS.CognitoIdentityProvider.initiate_auth(Blogex.AWS.client(), %{
AuthFlow: "USER_PASSWORD_AUTH",
AuthParameters: %{USERNAME: "bmalum_test", PASSWORD: "SecretPass123%$"},
ClientId: "gcb5gvidkg5temq7bp9t96m6p"
})
MFA Flow:
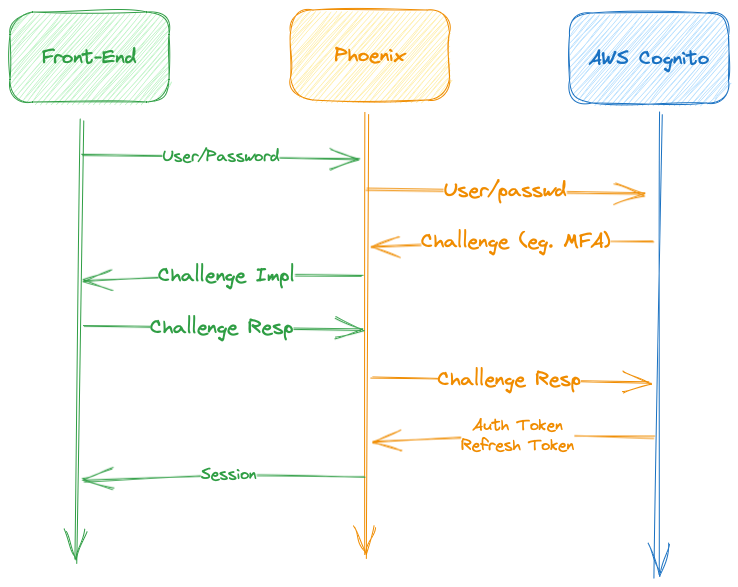
Next Up:
adding login with Apple & Google